Did you ever get stuck with the situation where you're triying to maintain all the state in the parent and child and all other sub-child are using that state values? In order to which you are passing the states as props through the parent to child and then to sub-child. This is a very common case with react apps, so you must have found it a bit uncomfortable to pass down props to deeper child and sub child levels.
Well, this is where Context API comes to play its role. Using this you can actually directly use the parent's state at deeper levels of child components without passing them down as props. Sounds interesting right ? Let's see things in action.
Let's take an example
Here we have a state at Parent.js
and we are passing state(name) and state setter (setName) down to ChildA.js
and SubChildA.js
.
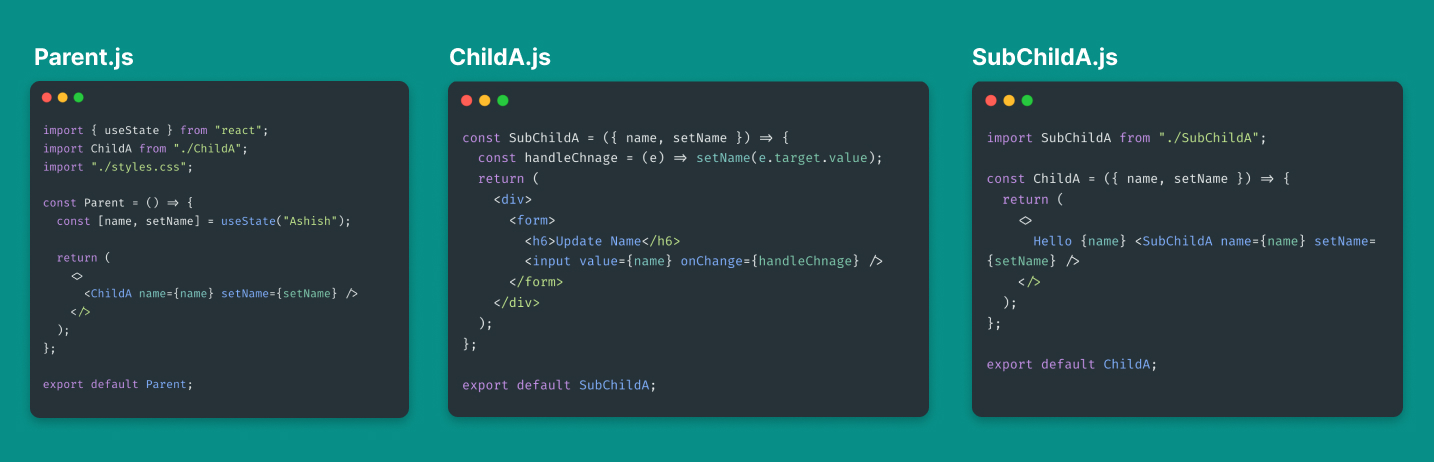
Context API
Now with context API, we will create a context of values that we wish to use as context anywhere in any of the child components in order to access the state without passing in as props.
So our first step would be to create a context in our Parent.js
. We can create context using createContext
. It takes the default value of the context as the parameter which in most cases we use an object with the key and default value like below.
export const StateContext = createContext({
name: "",
setName: ()=>{}
})
now here if you notice we have exported the StateContext which we will use later wherever we will need to use the context.
Now, in the second step, we need to provide our context to the chilld of Parent.js
for which we need to wrap all the children of Parent.js
with <StateContext.Provider></StateContext.Provider>
and we have to give it the actual values that we want to access at the deeper child components.
import { createContext, useState } from "react";
import ChildA from "./ChildA";
import "./styles.css";
export const StateContext = createContext({
name: "",
setName: () => {}
});
const Parent = () => {
const [name, setName] = useState("Ashish");
return (
<StateContext.Provider value={{ name, setName }}>
<ChildA />
</StateContext.Provider>
);
};
export default Parent;
Now, in the third step we have to use our context. This can be done using useContext
hook which will take the context (StateContext that we created) as the parameter and it will return the value sed by StateContext which can be used directly by the component.
import { useContext } from "react";
import { StateContext } from "./App";
import SubChildA from "./SubChildA";
const ChildA = () => {
const { name } = useContext(StateContext);
return (
<>
Hello {name} <SubChildA />
</>
);
};
export default ChildA;
So now this is what our components look like after implementing context. You would notice that now we are not passing down
name and setName
.
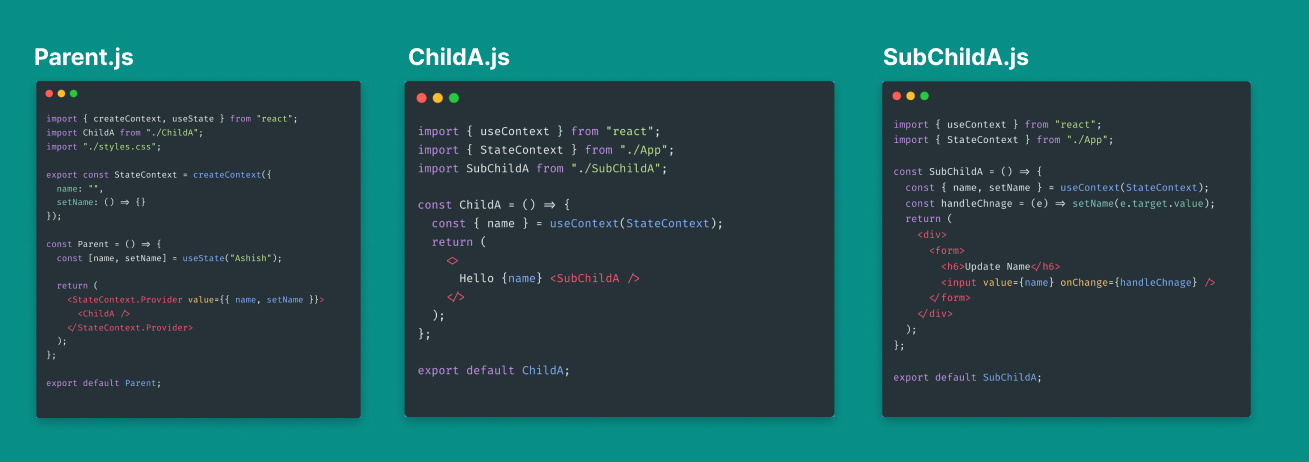
I hope this makes things easier for you to understand Context API. That's me signing off for today.